JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. is one of the most popular programming languages of the world.
Much of its popularity comes from its important role on the World Wide Web.
JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. joins the trifecta of HTMLHyperText Markup Language (HTML) is used to describe how web documents should be rendered by the web browser. When you navigate to a web page, the web server hosting that page serves your browser an HTML source file that is then rendered into the visible and interactive web page. and CSSCascading Stylesheets, or CSS, is a web technology that describes how the web page should look like and, up to a certain point, respond to interactions. If the HTML document defines the content, then CSS describes how that content should be structured, aligned, and painted in the browser. to provide the modern web experience, from creating dynamic web pages to running hybrid apps, from handling user interactions to running analytics collectors, and from being both the cause of and the solution to web performance issues.
From a programming perspective, JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. is something of a blank slate. Its roots are in the “C” family of languages (C, C++, Java…). However, it deviates enough from the stricter syntactic paradigms of these languages to make any type of comparisons counter-productive.
In this Topic, we’ll discuss some of JavaScript’s unique qualities from a technical marketing perspective. As such, we will barely scratch the surface of what can be done with JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript., especially in a full stack context.
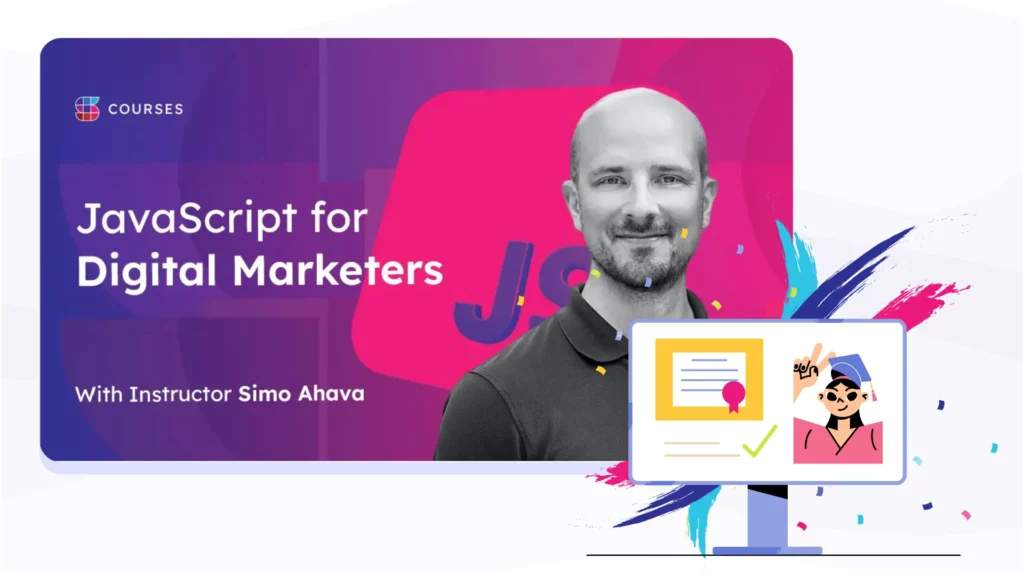
If you are interested in learning the language (it should be well within your capabilities as a technical marketer), you should take a look at the (free) JavaScript course on freeCodeCamp as well as Simmer’s course JavaScript For Digital Marketers.
JavaScript controls the browsing experience
As we discussed in the Web Browser Chapter, JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. is largely responsible for producing the interactive experiences of modern websites.
While HTMLHyperText Markup Language (HTML) is used to describe how web documents should be rendered by the web browser. When you navigate to a web page, the web server hosting that page serves your browser an HTML source file that is then rendered into the visible and interactive web page. and CSSCascading Stylesheets, or CSS, is a web technology that describes how the web page should look like and, up to a certain point, respond to interactions. If the HTML document defines the content, then CSS describes how that content should be structured, aligned, and painted in the browser. are the basis for the visible layout of the page, JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. enables the experience of the web page, including things like:
- Dynamically loading content upon user interaction
- Handling and validating forms
- Pop-ups and modals
- Animating and enriching ordinary interactions such as clicks and scrolls
JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. offers interfaces and APIsStrictly speaking application programming interfaces are methods and protocols in a piece of software that allow other sources to communicate with this software. More broadly they are used to describe any functions and methods that operate how the software works. for web developers to work with. With JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript., developers can assess, enrich, validate, and completely mutate web interactions that many users take for granted.
Example
Single-page appsA type of website where only the first page is loaded through a regular navigation action. The rest of the navigation pages are loaded dynamically with JavaScript without creating an actual navigation event in the browser. are entirely controlled by JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript.. When you click a link to navigate around the site, JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. blocks the link from redirecting the user to the linked page. Instead, the content is fetched dynamically from the web serverA machine connected to the World Wide Web, which is purpose-built to respond to HTTP requests from clients and for sending resources in response. and inserted on the page, yes, with JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript..
Often, JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. is used to enrich the page experience. Naturally, whether scripting is actually “enriching” instead of “irritating” really depends on how discreet it is.
Example
The tooltips in this Handbook have been engineered with JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript.. While there might have been a CSS-only way to do them, JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. gives more options for customization than a pure CSSCascading Stylesheets, or CSS, is a web technology that describes how the web page should look like and, up to a certain point, respond to interactions. If the HTML document defines the content, then CSS describes how that content should be structured, aligned, and painted in the browser. approach.
In addition to these examples, JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. is also the language of the digital marketing world. Analytics trackersSoftware that typically runs in the user's web browser or device, designed to collect data from the user to a server., advertising pixelsPixels are the smallest units that make up the visible part of a web page., page performance optimizations, and consentData protection laws often defer to positive consent for collecting or processing data from the user. Requesting consent usually involves a consent banner or a consent pop-up where the user is asked whether the site or app can collect data from them. management systems are all controlled (more or less) by JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript..
This proliferation of JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. is also problematic. JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. is often written in a very verbose way, and loading multiple files just to enrich the web experience can be detrimental to performance. For this reason, web browsers are slowly updating HTMLHyperText Markup Language (HTML) is used to describe how web documents should be rendered by the web browser. When you navigate to a web page, the web server hosting that page serves your browser an HTML source file that is then rendered into the visible and interactive web page. and CSSCascading Stylesheets, or CSS, is a web technology that describes how the web page should look like and, up to a certain point, respond to interactions. If the HTML document defines the content, then CSS describes how that content should be structured, aligned, and painted in the browser. to natively do some of the things that are today only enabled with JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript..
However, web standardsA set of agreed principles for how web browsers should render web content and how that web content can be interacted with. are very slow to evolve, and lack of cross-browser support means that as long as these updates to HTMLHyperText Markup Language (HTML) is used to describe how web documents should be rendered by the web browser. When you navigate to a web page, the web server hosting that page serves your browser an HTML source file that is then rendered into the visible and interactive web page. and CSSCascading Stylesheets, or CSS, is a web technology that describes how the web page should look like and, up to a certain point, respond to interactions. If the HTML document defines the content, then CSS describes how that content should be structured, aligned, and painted in the browser. are not widespread, JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. still needs to enable the same use cases for unsupported browsers.
Ready for a quick break?
We really recommend you take a small brain break at this point. After that, let’s jump into the weeds with some JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. under-the-hood content!
Document Object Model
The main interface between JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. and the web page is called the Document Object ModelThe dynamic, JavaScript-based representation of the page HTML and styles. When you look at a web page, you are actually looking at the visual layer of the DOM..
When the web browser rendersRendering happens when the web browser starts to convert the HTML document and its associated resources into the dynamic document the user sees and interacts with when visiting a web page. the HTMLHyperText Markup Language (HTML) is used to describe how web documents should be rendered by the web browser. When you navigate to a web page, the web server hosting that page serves your browser an HTML source file that is then rendered into the visible and interactive web page. source file, it turns it into a tree structure. In this structure, individual HTMLHyperText Markup Language (HTML) is used to describe how web documents should be rendered by the web browser. When you navigate to a web page, the web server hosting that page serves your browser an HTML source file that is then rendered into the visible and interactive web page. tagsNormally, tag references an HTML element (or node). In a marketing context, tags are used to denote HTML elements and JavaScript snippets specifically designed for collecting data to marketing vendors. become elements (also known as nodes) that can be nested within each other.
Each element in this tree is accessible with JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript.. You can use methods specifically designed for interacting with the DOMThe dynamic, JavaScript-based representation of the page HTML and styles. When you look at a web page, you are actually looking at the visual layer of the DOM., and these methods allow you to…
- Select elements on the page
- Create, modify, and delete elements on the page
- Add listeners to elements that react to user interactions and other on-page events
- Climb up and down the DOMThe dynamic, JavaScript-based representation of the page HTML and styles. When you look at a web page, you are actually looking at the visual layer of the DOM. as you look for a specific element
- Access the element’s content, attributes, properties, and other metadataMetadata is additional data about the data itself. For example, in an analytics system the "event" describes that action the user took, and metadata about the event could contain additional information about the user or the event itself.
If you look at this list and consider that every single thing a user sees or interacts with on a web page corresponds to a DOMThe dynamic, JavaScript-based representation of the page HTML and styles. When you look at a web page, you are actually looking at the visual layer of the DOM. element, you can understand how powerful JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. can be.
JavaScript’s ability to modify what the browser rendersRendering happens when the web browser starts to convert the HTML document and its associated resources into the dynamic document the user sees and interacts with when visiting a web page. from the HTMLHyperText Markup Language (HTML) is used to describe how web documents should be rendered by the web browser. When you navigate to a web page, the web server hosting that page serves your browser an HTML source file that is then rendered into the visible and interactive web page. source means that if you compare the page HTMLHyperText Markup Language (HTML) is used to describe how web documents should be rendered by the web browser. When you navigate to a web page, the web server hosting that page serves your browser an HTML source file that is then rendered into the visible and interactive web page. source file itself with what is actually shown on the page, the two might be completely different.
Especially with single-page appsA type of website where only the first page is loaded through a regular navigation action. The rest of the navigation pages are loaded dynamically with JavaScript without creating an actual navigation event in the browser., the web serverA machine connected to the World Wide Web, which is purpose-built to respond to HTTP requests from clients and for sending resources in response. only returns the initial HTMLHyperText Markup Language (HTML) is used to describe how web documents should be rendered by the web browser. When you navigate to a web page, the web server hosting that page serves your browser an HTML source file that is then rendered into the visible and interactive web page. source of the page load. As the user navigates the site, new content is loaded dynamically from the web serverA machine connected to the World Wide Web, which is purpose-built to respond to HTTP requests from clients and for sending resources in response., but the HTMLHyperText Markup Language (HTML) is used to describe how web documents should be rendered by the web browser. When you navigate to a web page, the web server hosting that page serves your browser an HTML source file that is then rendered into the visible and interactive web page. source of the page remains the same.
But even with “regular” sites, JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. can be used to heavily modify what was originally encoded in the page HTMLHyperText Markup Language (HTML) is used to describe how web documents should be rendered by the web browser. When you navigate to a web page, the web server hosting that page serves your browser an HTML source file that is then rendered into the visible and interactive web page..
Deep Dive
Google Tag Manager’s “magic” triggers
If you use a tag management systemTMS are software designed to create, manage, and deploy tags on web pages. They offer a user interface for managing the marketing tags on any given page. like Google TagNormally, tag references an HTML element (or node). In a marketing context, tags are used to denote HTML elements and JavaScript snippets specifically designed for collecting data to marketing vendors. Manager, you might have marvelled at how easy it is to create a “Click trigger” that automatically fires your tagsNormally, tag references an HTML element (or node). In a marketing context, tags are used to denote HTML elements and JavaScript snippets specifically designed for collecting data to marketing vendors. when the user clicks a specific element.
Well, under the hood GTM simply uses a DOMThe dynamic, JavaScript-based representation of the page HTML and styles. When you look at a web page, you are actually looking at the visual layer of the DOM. method to add a click listener to the web page, which then waits for the click event to happen. When the click happens, details about the event are inserted in to GTM’s Data LayerA globally available JavaScript structure on the web page designed to pass information between the web page and the tag management system., from where they can fire and provide data for your tagsNormally, tag references an HTML element (or node). In a marketing context, tags are used to denote HTML elements and JavaScript snippets specifically designed for collecting data to marketing vendors..
// Basic example of how GTM's click triggers work:
// 1. Add the listener to the web page, and run a callback function
// when the click happens.
document.addEventListener('click', function(e) {
// 2. Push details about the click target into dataLayer
window.dataLayer = window.dataLayer || [];
window.dataLayer.push({
event: 'gtm.click', // The name of the click event
'gtm.element': e.target, // The HTML element that was clicked
'gtm.elementId': e.target.id || '', // The value of the "id" attribute or empty string
'gtm.elementClasses': e.target.className || '', // The value of the "class" attribute or empty string
'gtm.elementUrl': e.target.href || e.target.action || '', // The target URL of the element, or empty string
});
});
When using Google TagNormally, tag references an HTML element (or node). In a marketing context, tags are used to denote HTML elements and JavaScript snippets specifically designed for collecting data to marketing vendors. Manager, it might seem like magic. But under the hood, GTM is simply interacting with JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. APIsStrictly speaking application programming interfaces are methods and protocols in a piece of software that allow other sources to communicate with this software. More broadly they are used to describe any functions and methods that operate how the software works. that would be available to you even without GTM.
Don’t miss this fact!
The Document Object ModelThe dynamic, JavaScript-based representation of the page HTML and styles. When you look at a web page, you are actually looking at the visual layer of the DOM. (DOMThe dynamic, JavaScript-based representation of the page HTML and styles. When you look at a web page, you are actually looking at the visual layer of the DOM.) is the main way of interacting with the web page using JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript.. As a technical marketer, the better you understand the machinations of the DOMThe dynamic, JavaScript-based representation of the page HTML and styles. When you look at a web page, you are actually looking at the visual layer of the DOM., the better you can anticipate how your work will be able to coexist with complicated web pages.
Dangers of types and scopes
Unruly usage of JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. on a web page can also be detrimental to the page experience.
As mentioned above, JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. can be used to delete elements on the page. For example, if you were to run this code on any web page, you would delete all the content of the page:
// Don't run this code on a web page, please
document.removeChild(document.querySelector('html'));
But you would probably never even unintentionally run a piece of code like that unless you were being manipulated by someone pulling a prank on you.
However, there are less insidious ways of JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. throwing a wrench into the works.
JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. is loosely typed. This means that if you declare a variableVariables are (usually small) pieces of code run in a TMS to fetch dynamic values for tags when they fire. A defining feature of variables is that they are re-evaluated whenever a tag fires. For example, if a variable fetches the exact time when a tag fired, it's important that it doesn't use the same, fixed value for all tags on the page., there’s no built-in mechanism that forces that variableVariables are (usually small) pieces of code run in a TMS to fetch dynamic values for tags when they fire. A defining feature of variables is that they are re-evaluated whenever a tag fires. For example, if a variable fetches the exact time when a tag fired, it's important that it doesn't use the same, fixed value for all tags on the page. to always have a certain type. This might lead you to inadvertently overwriting a variableVariables are (usually small) pieces of code run in a TMS to fetch dynamic values for tags when they fire. A defining feature of variables is that they are re-evaluated whenever a tag fires. For example, if a variable fetches the exact time when a tag fired, it's important that it doesn't use the same, fixed value for all tags on the page. with an unexpected type, which would then break any code that expects the type to be something specific.
For example, look at this:
// Google Tag Manager's dataLayer is defined as an array, as is expected.
window.dataLayer = [];
// Much later in the code, conflicting code overwrites dataLayer as a plain object.
window.dataLayer = {
pageType: 'article'
};
// Which then results in all of Google Tag Manager's own instruments breaking:
window.dataLayer.push({
event: 'pageChange'
});
// Uncaught TypeError: window.dataLayer.push is not a function
Because JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. doesn’t have type enforcement, nothing is stopping a programmer from rewriting the dataLayer
array (that GTM expects) into something else. But as it’s no longer an array, all the native array methods that GTM relies on (such as dataLayer.push
) fail because the overwritten dataLayer
is no longer an array.
Another problem with JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript., particularly in the context of the web, is that it has two main scopes: global and local.
Globally scoped variablesVariables are (usually small) pieces of code run in a TMS to fetch dynamic values for tags when they fire. A defining feature of variables is that they are re-evaluated whenever a tag fires. For example, if a variable fetches the exact time when a tag fired, it's important that it doesn't use the same, fixed value for all tags on the page. are available everywhere on the page. Any JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. running on the page can make use of them.
Locally scoped variablesVariables are (usually small) pieces of code run in a TMS to fetch dynamic values for tags when they fire. A defining feature of variables is that they are re-evaluated whenever a tag fires. For example, if a variable fetches the exact time when a tag fired, it's important that it doesn't use the same, fixed value for all tags on the page. are only available in the function context where they are declared.
There’s a saying among JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. web developers: “Don’t pollute the global scope“. This means that unless absolutely necessary, always declare variablesVariables are (usually small) pieces of code run in a TMS to fetch dynamic values for tags when they fire. A defining feature of variables is that they are re-evaluated whenever a tag fires. For example, if a variable fetches the exact time when a tag fired, it's important that it doesn't use the same, fixed value for all tags on the page. locally.
Why? Because sometimes when you have a scenario where a local variableVariables are (usually small) pieces of code run in a TMS to fetch dynamic values for tags when they fire. A defining feature of variables is that they are re-evaluated whenever a tag fires. For example, if a variable fetches the exact time when a tag fired, it's important that it doesn't use the same, fixed value for all tags on the page. would suffice and you instead use the global scope, you might end up overwriting the original, globally scoped variableVariables are (usually small) pieces of code run in a TMS to fetch dynamic values for tags when they fire. A defining feature of variables is that they are re-evaluated whenever a tag fires. For example, if a variable fetches the exact time when a tag fired, it's important that it doesn't use the same, fixed value for all tags on the page..
// dataLayer is declared globally:
window.dataLayer = [];
// A function creates local scope:
function loadPage() {
console.log('Page is loaded');
// BAD! This overwrites the globally scoped dataLayer:
dataLayer = {
pageType: 'loaded'
};
// BETTER! The "var" keyword creates a new dataLayer variable that is locally scoped:
var dataLayer = {
pageType: 'loaded'
};
// BEST! This creates a locally scoped variable that doesn't have any conflict with the well-known dataLayer name:
var customData = {
pageType: 'loaded'
};
}
The key takeaway here is that if you want to start producing custom code on web page, you need to be aware of these two quirks and the plethora of other JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. idiosyncrasies that might show up and decimate the entire browsing experience of your website.
This isn’t meant to scare you away from using JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript.. But as a technical marketer, you might find yourself frequently offering code snippets and issuing requests for modifying on-page JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript.. In these cases, you should be aware of best practices when it comes to coding, such as those that are extensively covered in Simmer’s JavaScript course.
Don’t miss this fact!
JavaScript’s relaxed approach to types and its division of global and local scope can really trip you up if you want to produce code on the site but don’t understand these concepts.
Debug JavaScript on the page
If you ever need to test, debug, or validate JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. on a web page, you need to familiarize yourself with the browser’s developer toolsBrowsers offer comprehensive toolkits for debugging many different aspects of the modern web experience. Learning these tools is one of the most important things for a technical marketer..
Google Chrome, for example, has an extremely powerful set of developer toolsBrowsers offer comprehensive toolkits for debugging many different aspects of the modern web experience. Learning these tools is one of the most important things for a technical marketer. that you can open by pressing the F12 key on your keyboard.
For JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. debugging purposes, you’ll find the Elements panel and the Console panel most useful.
The Elements panel is where you can see the DOMThe dynamic, JavaScript-based representation of the page HTML and styles. When you look at a web page, you are actually looking at the visual layer of the DOM. in all its glory. You can search for elements, you can expand and contract the tree’s nodes, you can delete elements, you can edit them, and you can add new elements – all in the developer tools’ Elements panel without having to write a single line of JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript..
When debugging your JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript., the Elements panel can quickly ascertain whether a certain element exists on the page, for example. If you are trying to write code that relies on the existence of an element, it’s good to verify it actually does exist with this panel.
In the Elements panel, you can also edit the styles of any given element on the page, which is very useful if you want to test alternative approaches to styling your content.
The Console panel is your best JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. debugging device, however. It lets you run any browser JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. in the context of the current page.
If you have code you want to test before running it through Google TagNormally, tag references an HTML element (or node). In a marketing context, tags are used to denote HTML elements and JavaScript snippets specifically designed for collecting data to marketing vendors. Manager, for example, executing it in the browser’s JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. console is a great way to make sure it works as intended.
Any code you run in the JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. console can interact with the DOMThe dynamic, JavaScript-based representation of the page HTML and styles. When you look at a web page, you are actually looking at the visual layer of the DOM. in its current state. If the elements on the page change, you can run the code again and it will react with the updated state.
As a technical marketer, getting acquainted with the browser’s developer toolsBrowsers offer comprehensive toolkits for debugging many different aspects of the modern web experience. Learning these tools is one of the most important things for a technical marketer. is a huge service to your professionalism.
You’ll be able to test, debug, and verify the impact of your marketing efforts before you need to escalate them to IT. You can execute your own code, update the source files of the site, and run full performance profiling tests on the web pages through your own, local browser environment.
At the same time, being an expert of developer toolsBrowsers offer comprehensive toolkits for debugging many different aspects of the modern web experience. Learning these tools is one of the most important things for a technical marketer. only takes you so far. In the context of this Topic, your main job is to be aware of the devastating power and limitless capability of JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript.. While you don’t need to know how to code a single line of JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. to be an adequate technical marketer, learning at least the basics of the language will go a long way into helping you break through professional plateaus and into opening new career paths for you.
Key takeaway #1: JavaScript is the language of the web browser
JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. is the third component to the trifecta of web design, complementing HTMLHyperText Markup Language (HTML) is used to describe how web documents should be rendered by the web browser. When you navigate to a web page, the web server hosting that page serves your browser an HTML source file that is then rendered into the visible and interactive web page. and CSSCascading Stylesheets, or CSS, is a web technology that describes how the web page should look like and, up to a certain point, respond to interactions. If the HTML document defines the content, then CSS describes how that content should be structured, aligned, and painted in the browser. as the language that enables the experience of the web page. When you interact with a web page in the browser, these interactions are fuelled by JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript.. Things like dynamically loading content, form validation, pop-ups, modals, and animated clicks and scrolls are (almost always) generated by JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript.. For a technical marketer, JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. is present in almost every aspect of their job, as the web browser is still the most important tool in the marketer’s arsenal.
Key takeaway #2: DOM is the dynamic representation of the web page
For a technical marketer, the most important piece of browser JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. to understand is the Document Object ModelThe dynamic, JavaScript-based representation of the page HTML and styles. When you look at a web page, you are actually looking at the visual layer of the DOM. (DOMThe dynamic, JavaScript-based representation of the page HTML and styles. When you look at a web page, you are actually looking at the visual layer of the DOM.). It’s generated by the web browser from the source HTMLHyperText Markup Language (HTML) is used to describe how web documents should be rendered by the web browser. When you navigate to a web page, the web server hosting that page serves your browser an HTML source file that is then rendered into the visible and interactive web page. and its linked resources (CSSCascading Stylesheets, or CSS, is a web technology that describes how the web page should look like and, up to a certain point, respond to interactions. If the HTML document defines the content, then CSS describes how that content should be structured, aligned, and painted in the browser., images, scripts…). It’s represented by a node tree, where individual HTMLHyperText Markup Language (HTML) is used to describe how web documents should be rendered by the web browser. When you navigate to a web page, the web server hosting that page serves your browser an HTML source file that is then rendered into the visible and interactive web page. elements are all part of a nested tree structure. These elements, their position, and their individual styles can be manipulated with JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript.. This is what enables the modern web browsing experience.
Key takeaway #3: Global and local scope
In addition to the DOMThe dynamic, JavaScript-based representation of the page HTML and styles. When you look at a web page, you are actually looking at the visual layer of the DOM., another key aspect of JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. is the notion of “scope”. Fundamentally, JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. operates in two scopes: global and local. Global scope means that the variablesVariables are (usually small) pieces of code run in a TMS to fetch dynamic values for tags when they fire. A defining feature of variables is that they are re-evaluated whenever a tag fires. For example, if a variable fetches the exact time when a tag fired, it's important that it doesn't use the same, fixed value for all tags on the page. and functions you declare are available globally – in any part of any JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. code running on the page. Local scope means that these are only available within the immediate context. Writing JavaScriptJavaScript is the main language of the dynamic web. The web browser renders the HTML source file into a dynamic document that can be interacted with using JavaScript. in global scope is dangerous, because you risk overwriting similar variablesVariables are (usually small) pieces of code run in a TMS to fetch dynamic values for tags when they fire. A defining feature of variables is that they are re-evaluated whenever a tag fires. For example, if a variable fetches the exact time when a tag fired, it's important that it doesn't use the same, fixed value for all tags on the page. or functions that were added to global scope by some other piece of code.